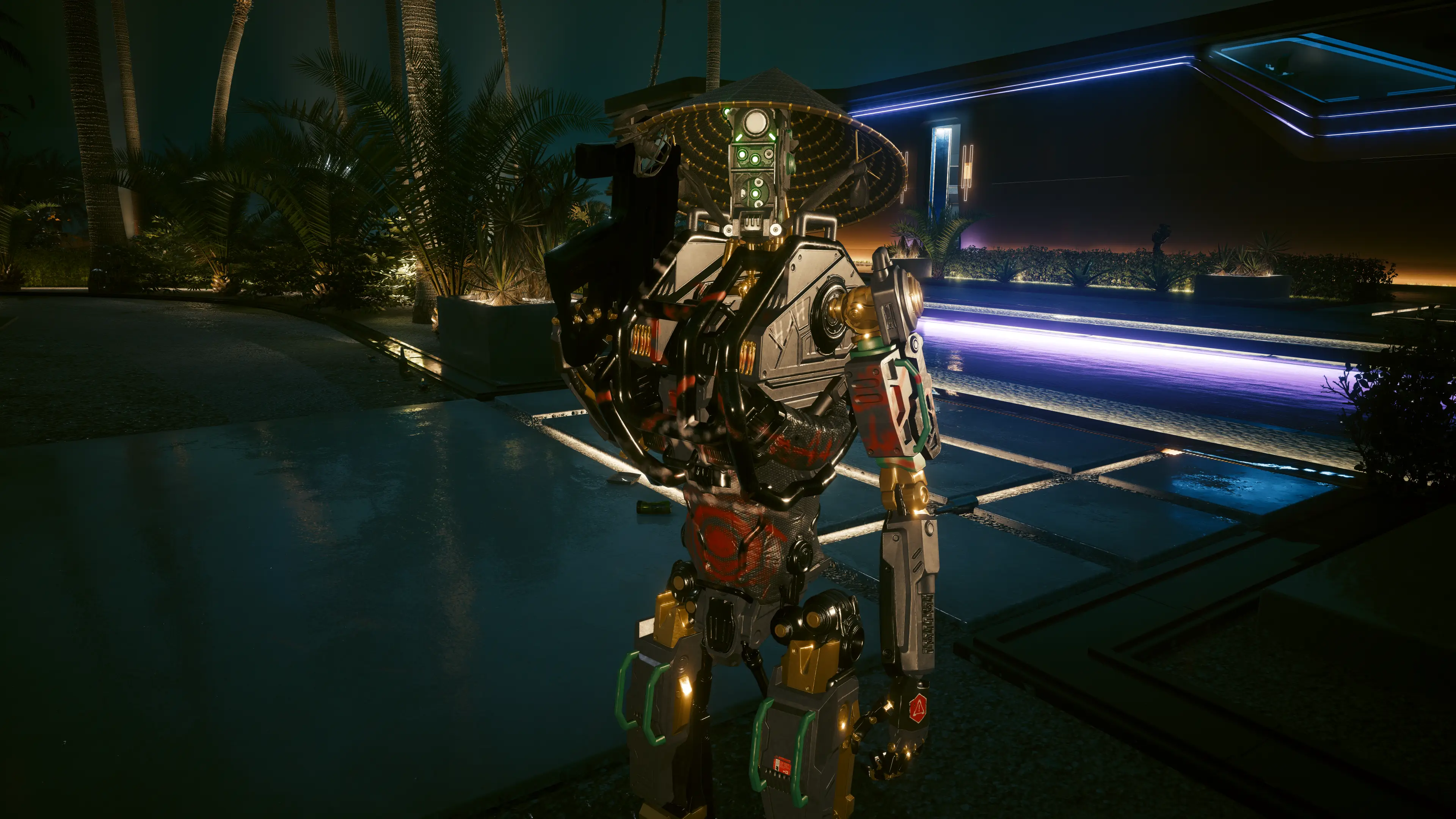
Raspberry Pi Cluster Monitor Project
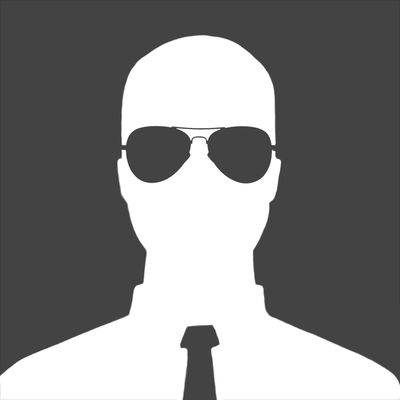
Created
11:27 Sunday 8th September 2024
Updated
14:18 Thursday 24th April 2025
With an ever-expanding roster of raspberry pi's getting added to my local cluster it became practical to monitor them in a single place. What better way to do that with another raspberry pi wired up to a 7' touchscreen with a visual status app.
What do we want to do?
The goal of this application is to display system information of a number of raspberry pi computers. To achevie this we'll need 2 seperate repos:
- A node script that runs on each computer we wish to report on, it grabs the system information and exposes it via an express driven api
- A React driven web application that queries each of the raspberry pi APIs and displays the information they provide
Starting with the API that is going to query all of the system info we need and expose it via an API, here is the node.js code that is going to provide that:
import express from "express"; import { RaspberryPiInfo } from "@alexkratky/rpi-info"; import { getCpuTemperature, getGpuTemperature } from "raspberrypi-sys-info"; import checkDiskSpace from "check-disk-space"; import memory from "node-free"; const app = express(); const port = 7777; const raspberryPiInfo = new RaspberryPiInfo(); const raspiModelInfo = raspberryPiInfo.detect(); async function displaySystemInfo() { try { const cpuTemp = await getCpuTemperature(); const gpuTemp = await getGpuTemperature(); return { cpuTemp: cpuTemp, gpuTemp: gpuTemp, }; } catch (error) { console.error(error.message); return { cpuTemp: null, gpuTemp: null, }; } } app.use(express.json()); app.get("/", async (req, res) => { const systemStats = await displaySystemInfo(); let diskSpace; await checkDiskSpace("/").then((space) => { diskSpace = { diskPath: space.diskPath, diskSize: space.size, diskFree: space.free, }; }); const memTotal = memory.total(); const memUsed = memory.used(); const memFree = memory.free(); const memoryStats = { memTotal, memUsed, memFree, }; const result = { ...raspiModelInfo, ...systemStats, ...diskSpace, ...memoryStats, }; res.status(200).json(result); }); app.listen(port, () => console.log("`Example app listening on port port" + port) );